There are 4 different types of loop which we can use in verilog – the for loop, while loop, forever loop and repeat loop.
Forever loop
two different formats:
forever sentence;
//or
forever begin
multiple sentences;
end
one of the most common use cases for the forever loop is generating a clock signal in a verilog test bench.
Repeat loop
We use the repeat loop to execute a given block of verilog code a fixed number of times. We specify the number of times the code block will execute in the repeat loop declaration.
two different formats:
repeat(expression) sentence;
//or
repeat(expression) begin
multiple sentences;
end
example: Multiplier
parameter size = 8, longsize = 16;
reg[size:1] opa, opb;
reg[longsize:1] result;
begin:mult
reg[longsize:1] shift_opa, shift_opb;
shift_opa = opa;
shift_opb = opb;
result = 0;
repeat(size)
begin
if(shift_opb[1])
result = result+shift_opa;
shift_opa = shift_opa <<1;
shift_opb = shift_opb >>1;
end
end
While loop
We can think of the while loop as an if statement that executes repeatedly. The specified condition is evaluated before each iteration of the loop.
two different formats:
while(expression) sentence;
//or
while(expression) begin
multiple sentences;
end
example: use while loop to count 1 in the 8 -bit binary value
begin: count1s
reg[7:0] tempreg;
count = 0;
tempreg = rega;
while(tempreg)
begin
if(tempreg[0]) count = count + 1;
tempreg = tempreg >> 1;
end
end
For loop
general form:
for(<initial_condition>; <stop_condition>; <increment>)
We use the <initial_condition> field to set the initial value of our loop variable. We must declare the variable that we use in our loop before we can use it in our code.
The <stop_condition> field is the conditional statement which determines how many times the loop runs. The for loop will continue to execute until this field evaluates as false.
We use the <increment> field to determine how the loop variable is updated in every iteration of the loop.
its basic functions
step1: calculate initial_condition
step2: calculate stop_condition, if it is true(no 0), then execute codes inside for loop. Then execute step 3. If the outcome of step 3 if false(0), then end loop and jump to step 5.
step3: if the stop_condition is true, after executing codes, calculate increment.
step4: return to step 2
step5: end for loop and continue running codes down below.
Example: using for loop to achieve Multiplier
//use for loop to initialize memory
begin: init_mem
reg[7:0] tempi;
for(tempi=0;tempi<memsize;tempi=tempi+1)
memory[tempi]=0;
end
//use for loop to achieve Multiplier
parameter size = 8, longsize = 16;
reg[size:1] opa,opb;
reg[longsize:1] result;
begin: mult
integer bindex;
result = 0;
for(bindex = 1;bindex <= size; bindex = bindex +1)
if(opb[bindex])
result = result + (opa<<(bindex-1));
end
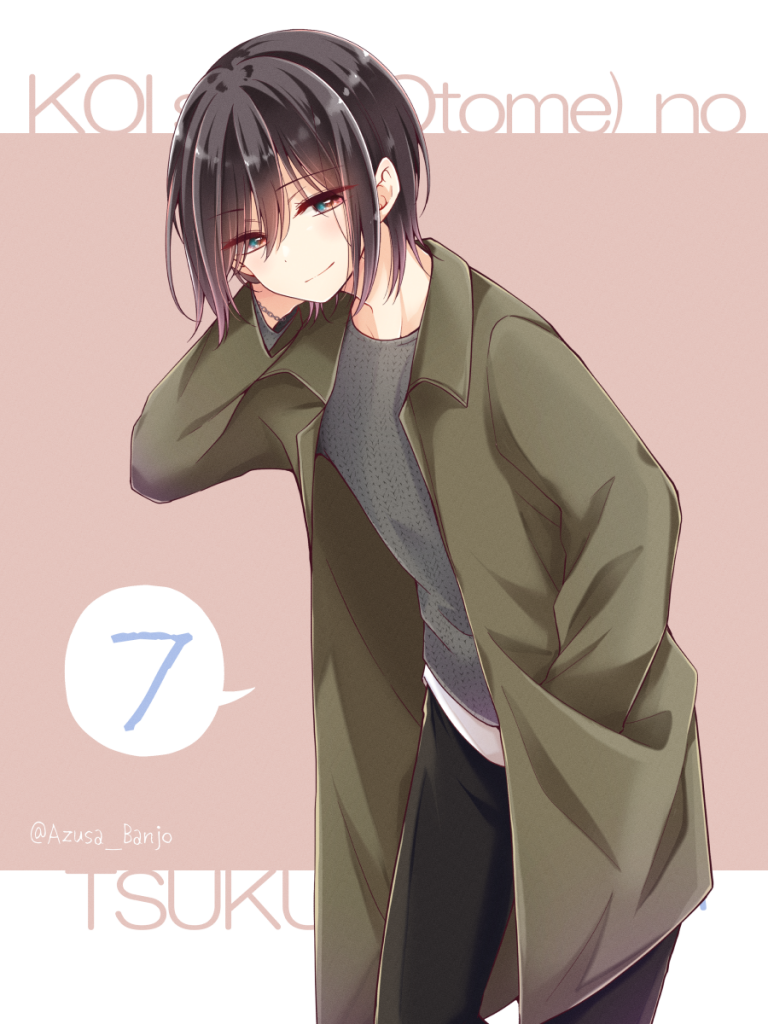