知识点1 STL的概述
STL(Standard Template Library)标准模板库
STL的三大组件:容器(container)、算法(algorithm)、迭代器(iterator)
算法用来操作数据、容器用来存储数据。算法不依赖于数据。在算法和容器之间引入迭代器的概念。每一个容器都会有相应的迭代器。算法就可以借助迭代器操作容器。
STL的六大组件:容器 算法 迭代器 仿函数 适配器 空间配置器
容器:容器,置物之所也。存放数据
算法:算法,问题之解法也。操作数据
迭代器:容器和算法的桥梁,是一种抽象的设计概念,现实程序语言中没有直接对应这个概念的实物。迭代器提供一种方法使之能够寻访某个容器所含的各个元素,而又无需暴露该容器内部表示方式。是STL的关键所在。
仿函数:为算法提供更多的策略
适配器:为算法提供更多的参数接口
空间配置器:管理容器和算法的所需配置空间
算法的分类:质变算法:运算过程中会更改区间内的元素的内容。例如拷贝,构造,删除等
非质变算法:运算过程中不会改变区间内元素的内容。如查找,计数,遍历等
迭代器的种类
输入迭代 器 | 提供对数据的只读的访问 | 只读,支持++、==、!= |
输出迭代器 | 提供对数据的只写访问 | 只写,支持++ |
前向迭代器 | 提供读写操作,能向前推进迭代器 | 读写,支持++、==、!= |
双向迭代器 | 提供读写操作,能向前向后操作 | 读写,支持++、-- |
随机访问迭代器 | 提供读写操作,并能以跳跃方式访问容器的任意数据,是功能最强大的迭代器 | 读写,支持++、--、[n]、-n、<、<=、>、>= |
知识点2迭代器的案例
#include <iostream>
#include <vector>
#include <algorithm>
using namespace std;
void myPrint(int val)
{
cout << val << " ";
}
int main()
{
vector <int> v;
v.push_back(100);
v.push_back(200);
v.push_back(300);
v.push_back(400);
vector<int>::iterator beginIt = v.begin();
vector<int>::iterator endIt = v.end();
//for循环遍历1
for (;beginIt != endIt; beginIt++)
{
cout << *beginIt << " ";
}
cout << endl;
//for循环遍历2
for(vector<int>::iterator it = v.begin(); it != v.end(); it++)
{
cout << *it << " ";
}
cout << endl;
//STL 提供的算法来遍历容器 #include <algorithm>
//for_each 从容器的起始到结束逐个元素取出
for_each(v.begin(), v.end(),myPrint);
cout << endl;
return 0;
}
容器也可以存放自定义数据类型
#include <iostream>
#include <vector>
#include <algorithm>
#include <cstring>
using namespace std;
class Person
{
public:
string name;
int age;
Person(string name, int age)
{
this->name = name;
this->age = age;
}
};
void myPrintPerson(Person &ob)
{
cout << "name:" << ob.name << ", age:" << ob.age << endl;
}
int main()
{
Person ob1("阿尔贝尔",114514);
Person ob2("小仓唯",110);
Person ob3("里昂",23);
Person ob4("耗子尾汁",69);
vector<Person> v;
v.push_back(ob1);
v.push_back(ob2);
v.push_back(ob3);
v.push_back(ob4);
for_each(v.begin(), v.end(), myPrintPerson);
return 0;
}
容器也可以嵌套容器
#include <iostream>
#include <vector>
#include <algorithm>
#include <cstring>
using namespace std;
int main()
{
vector<int> v1;
vector<int> v2;
vector<int> v3;
v1.push_back(10);
v1.push_back(20);
v1.push_back(30);
v1.push_back(40);
v2.push_back(100);
v2.push_back(200);
v2.push_back(300);
v2.push_back(400);
v3.push_back(1000);
v3.push_back(2000);
v3.push_back(3000);
v3.push_back(4000);
//再定义一个vector容器存放v1 v2 v3
vector<vector<int>> v;
v.push_back(v1);
v.push_back(v2);
v.push_back(v3);
//for循环遍历
for(vector<vector<int>>::iterator it = v.begin(); it!= v.end(); it++)
{
for(vector<int>::iterator mit = (*it).begin(); mit!= (*it).end(); mit++)
{
cout << *mit << " ";
}
cout << endl;
}
cout << endl;
return 0;
}
知识点3 string类
string 的构造和赋值
#include <iostream>
#include <cstring>
using namespace std;
int main()
{
//使用字符串s初始化
string str1("hello string");
cout << str1 << endl;//hello string
//使用n个字符串c初始化
string str2(10,'H');
cout << str2 << endl;//HHHHHHHHHH
string str3 = str2;
cout << str3 << endl;//HHHHHHHHHH
string str4;
str4 = str1;
cout << str4 << endl;//hello string
string str5;
str5 = "hello str5";
cout << str5 << endl;//hello str5
string str6;
str6 = 'H';
cout << str6 << endl;//H
string str7;
str7.assign("hello str7");
cout << str7 << endl;//hello str7
string str8;
str8.assign("hello str7",5);
cout << str8 << endl;//hello
string str9;
str9.assign(str8);
cout << str9 << endl;////hello
string str10;
str10.assign(10,'W');
cout << str10 << endl;//WWWWWWWWWW
string str11;
str11.assign("momoka",2,2);
cout << str11 << endl;//mo
return 0;
}
string的字符的存取
#include <iostream>
#include <cstring>
#include <exception>
using namespace std;
int main()
{
string str1 = "hello string";
cout << str1[1] << endl;
cout << str1.at(1) << endl;
str1[1] = 'E';
cout << str1 << endl;
str1.at(7) = 'T';
cout << str1 << endl;
//[]和at()的区别
try
{
//str1[1000] = 'G';//越界不抛出异常
str1.at(1000) = 'G';//basic_string::at: __n (which is 1000) >= this->size() (which is 12)
}
catch (exception &e)
{
cout << e.what() << endl;
}
return 0;
}
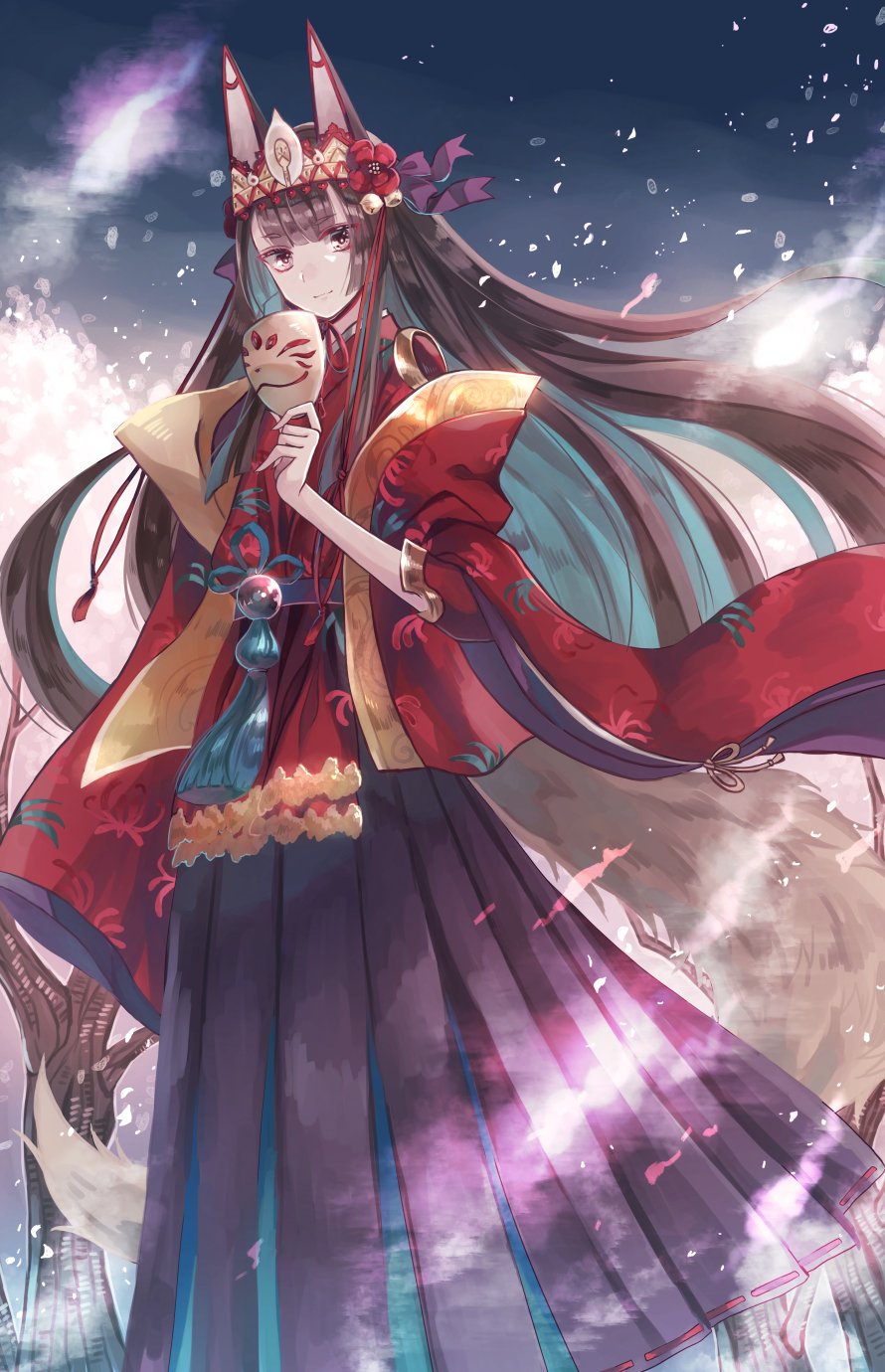