类模板的应用源代码
设计一个数组模板类,完成对不同类型数组的管理
myArray.hpp
#ifndef MYARRAY_HPP_INCLUDED
#define MYARRAY_HPP_INCLUDED
#include <iostream>
using namespace std;
template<class T>
class MyArray
{
private:
T *addr;
int capacity;
int size;
public:
MyArray(int capacity);
MyArray(const MyArray &ob);
~MyArray();
//尾插法
void push_back(const T &val);
//遍历数组
void printArray(void);
};
template<class T>
MyArray<T>::MyArray(int capacity)
{
//对于 自定义数据类型 new person[10] 数组中的每个元素 都会调用无参构造
this->addr = new T[capacity];
this->capacity = capacity;
this->size = 0;
}
template<class T>
MyArray<T>::MyArray(const MyArray &ob)
{
this->capacity = ob.capacity;
this->addr = new T[this->capacity];
int i = 0;
for(i = 0;i<ob.size;i++)
{
this->addr[i] =ob.addr[i];
}
this->size = ob.size;
}
template<class T>
MyArray<T>::~MyArray()
{
if(this->addr != NULL)
{
delete[] this->addr;
this->addr = NULL;
}
}
template<class T>
void MyArray<T>::push_back(const T &val)
{
//数组的实际个数size不能超过capacity
if(this->size == this->capacity)
{
cout << "容器已满" << endl;
return;
}
this->addr[this->size] = val;
this->size++;
}
template<class T>
void MyArray<T>::printArray(void)
{
int i = 0;
for(i=0;i<this->size;i++)
{
//如果输出的是自定义数据必须重载运算符
cout << this->addr[i] << " ";
}
cout << endl;
}
#endif // MYARRAY_HPP_INCLUDED
main.cpp
#include <iostream>
#include"myArray.hpp"
#include <cstring>
using namespace std;
class Person
{
friend ostream& operator<<(ostream &out, const Person&ob);
private:
string name;
int age;
public:
Person ()
{
}
Person(string name, int age)
{
this->name = name;
this->age = age;
}
};
ostream& operator<<(ostream &out, const Person&ob)
{
out << "name = " << ob.name << ", age = " << ob.age << endl;
return out;
}
int main()
{
MyArray<char> ob(10);
ob.push_back('a');
ob.push_back('b');
ob.push_back('c');
ob.push_back('d');
ob.printArray();
MyArray<int> ob2(10);
ob2.push_back(10);
ob2.push_back(20);
ob2.push_back(30);
ob2.push_back(40);
ob2.printArray();
//存放自定义数据
MyArray<Person> ob3(10);
ob3.push_back(Person ("阿尔贝尔",114514));
ob3.push_back(Person ("库鲁特",22222));
ob3.push_back(Person ("吱吱吱",678));
ob3.push_back(Person ("帮大忙了",0));
ob3.printArray();
return 0;
}
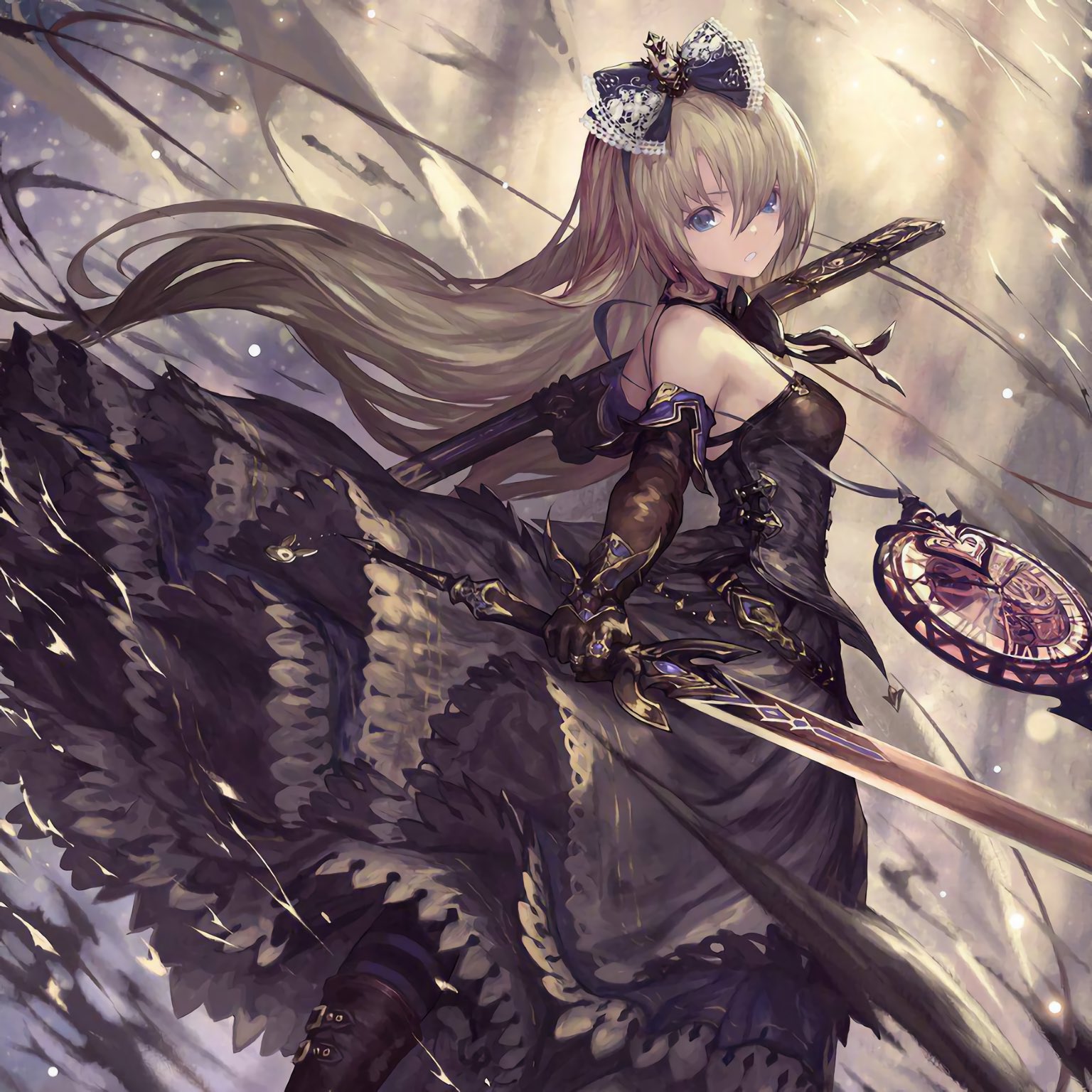
“C++笔记6.00”的一个回复