知识点4 重载=运算符(重要)
类中没有指针成员时,不需要重载=运算符(使用默认的浅拷贝就可以完成)
如果类中有了指针成员,那就必须重载=运算符,且自定义拷贝构造函数
#include <iostream>
#include <cstring>
using namespace std;
class Person
{
private:
char *name;
public:
Person()
{
name = NULL;
cout << "无参构造" << endl;
}
Person(char *name)
{
this->name = new char[strlen(name) + 1];
strcpy(this->name,name);
cout << "有参构造" << endl;
}
~Person()
{
cout << "析构函数" << endl;
if(this->name != NULL)
{
delete [] this->name;
this->name = NULL;
}
}
void showPerson(void)
{
cout << "name = " << name << endl;
}
Person(const Person &ob)//ob代表的旧对象
{
cout << "拷贝构造函数" << endl;
this->name = new char[strlen(ob.name) + 1];
strcpy(this->name,ob.name);
}
Person& operator=(Person &ob)
{
if (this->name != NULL)//清空以前的赋值
{
delete []this->name;
this->name = NULL;
}
cout << "运算符重载" << endl;
this->name = new char[strlen(ob.name) + 1];
strcpy(this->name,ob.name);
return *this;
}
};
int main()
{
Person ob1("momoka");
ob1.showPerson();
Person ob2 = ob1;//调用拷贝构造
Person ob3;
Person ob4;
ob4 = ob3 = ob1;//重载=运算符
return 0;
}
知识点5 重载等于和不等于(==、!=)
bool operator==(Person &ob)
{
if(strcmp(this->name,ob.name) == 0)
{
return true;
}
return false;
}
bool operator!=(Person &ob)
{
if(strcmp(this->name,ob.name) != 0)
{
return true;
}
return false;
}
知识点6 函数调用符()的重载以及仿函数的定义
#include <iostream>
using namespace std;
class Fun
{
public:
int my_add(int x, int y)
{
return x + y;
}
//第一个()是重载的符号,第二个()是标明传递的参数
int operator()(int x, int y)
{
return x + y;
}
};
int main()
{
Fun fun;
cout << fun.my_add(100,200) << endl;
cout << fun.operator() (100,200) << endl;
cout << fun(100,200) << endl;
//此处不是一个真正的函数,是一个对象名和()结合,调用重载运算符,称为仿函数
cout << Fun()(100,200) << endl;//声明了匿名对象
return 0;
}
知识点7 不要重载&&和||
原因:用户无法实现&&和||的短路特性
A && B如果A是假的,B就不会执行
A || B 如果A是真的,B就不会执行
知识点8 符号重载的总结
运算符 | 建议使用 |
所有的一元运算符 | 成员 |
= () [] -> * | 必须是成员 |
+= -= /= *= ^= &= != %= >>= <<= == | 成员 |
其他二元运算符 << >> | 非成员 |
知识点9 强化训练字符串类String
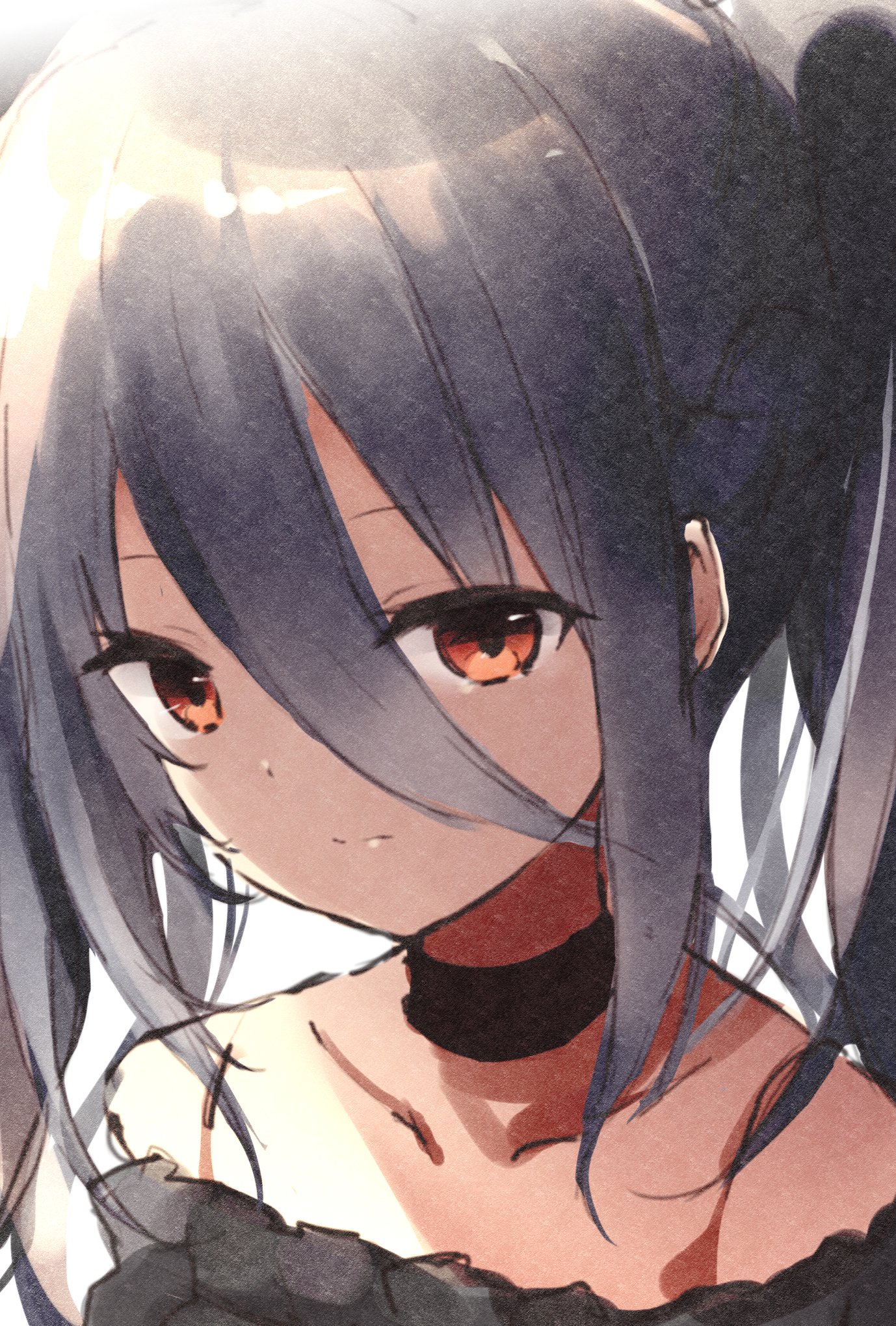