封装一个电视机的类
#include <iostream>
using namespace std;
class TV
{
friend class Remote;
private :
int mState;//电视状态,开机,还是关机
int mVolume;//电视机音量
int mChannel;//电视频道
public :
enum{On,Off}; //电视机状态
enum{minVol ,maxVol = 100}; //音量从1到100
enum{minChannel = 1, maxChannel = 255};//频道从1到255
TV()
{
this->mState = Off;//默认关机
this->mVolume = minVol;
this->mChannel = minChannel;
}
//电源按钮
void onOrOff()
{
this->mState = (this->mState == On ? Off : On);
}
void volumeUp(void)
{
if(this->mVolume >= maxVol)
{
cout << "最大音量!" << endl;
return;
}
else if (this ->mState == Off)
{
cout << "未打开电源!" << endl;
return;
}
this->mVolume++;
}
void volumeDown(void)
{
if(this->mVolume <= minVol)
{
cout << "最小音量!" << endl;
return;
}
else if (this ->mState == Off)
{
cout << "未打开电源!" << endl;
return;
}
this->mVolume--;
}
void channelUp(void)
{
if(this->mChannel >= maxChannel)
{
this->mChannel = minChannel;
}
else if (this ->mState == Off)
{
cout << "未打开电源!" << endl;
return;
}
this->mChannel++;
}
void channelDown(void)
{
if(this->mChannel <= minChannel)
{
this->mChannel = maxChannel;
}
else if (this ->mState == Off)
{
cout << "未打开电源!" << endl;
return;
}
this->mChannel--;
}
void showTVState(void)
{
cout << "电视机的状态为:" << (this->mState==On?"开机":"关机") << endl;
cout << "电视机的音量:" << this->mVolume << endl;
cout << "电视机的频道:" << this->mChannel << endl;
}
~TV()
{
cout << "析构函数" << endl;
}
};
//遥控器的类
class Remote
{
private:
TV *pTv;
public:
Remote(TV *pTv )
{
this->pTv = pTv;
}
void volumeUp(void)
{
this->pTv->volumeUp();
}
void volumeDown(void)
{
this->pTv->volumeDown();
}
void channelUp(void)
{
this->pTv->channelUp();
}
void channelDown(void)
{
this->pTv->channelDown();
}
void onOrOff(void)
{
this->pTv->onOrOff();
}
void setChannel(int num)
{
if(num >=TV::minChannel && num <= TV::maxChannel && this->pTv->mState == TV::On)
{
this->pTv->mChannel = num;
}
}
void showTVState(void)
{
this->pTv->showTVState();
}
};
int main()
{
TV tv;
Remote remote(&tv);
//插电开机
tv.onOrOff();
//音量从0上调4次
tv.volumeUp();
tv.volumeUp();
tv.volumeUp();
tv.volumeUp();
//频道从1上调3次
tv.channelUp();
tv.channelUp();
tv.channelUp();
//显示状态
tv.showTVState();
//用遥控器关机
remote.onOrOff();
remote.channelUp();
remote.volumeUp();
//显示状态
tv.showTVState();
//用遥控器开机控制
remote.onOrOff();
remote.volumeDown();
remote.volumeDown();
remote.setChannel(123);
//显示状态
tv.showTVState();
return 0;
}
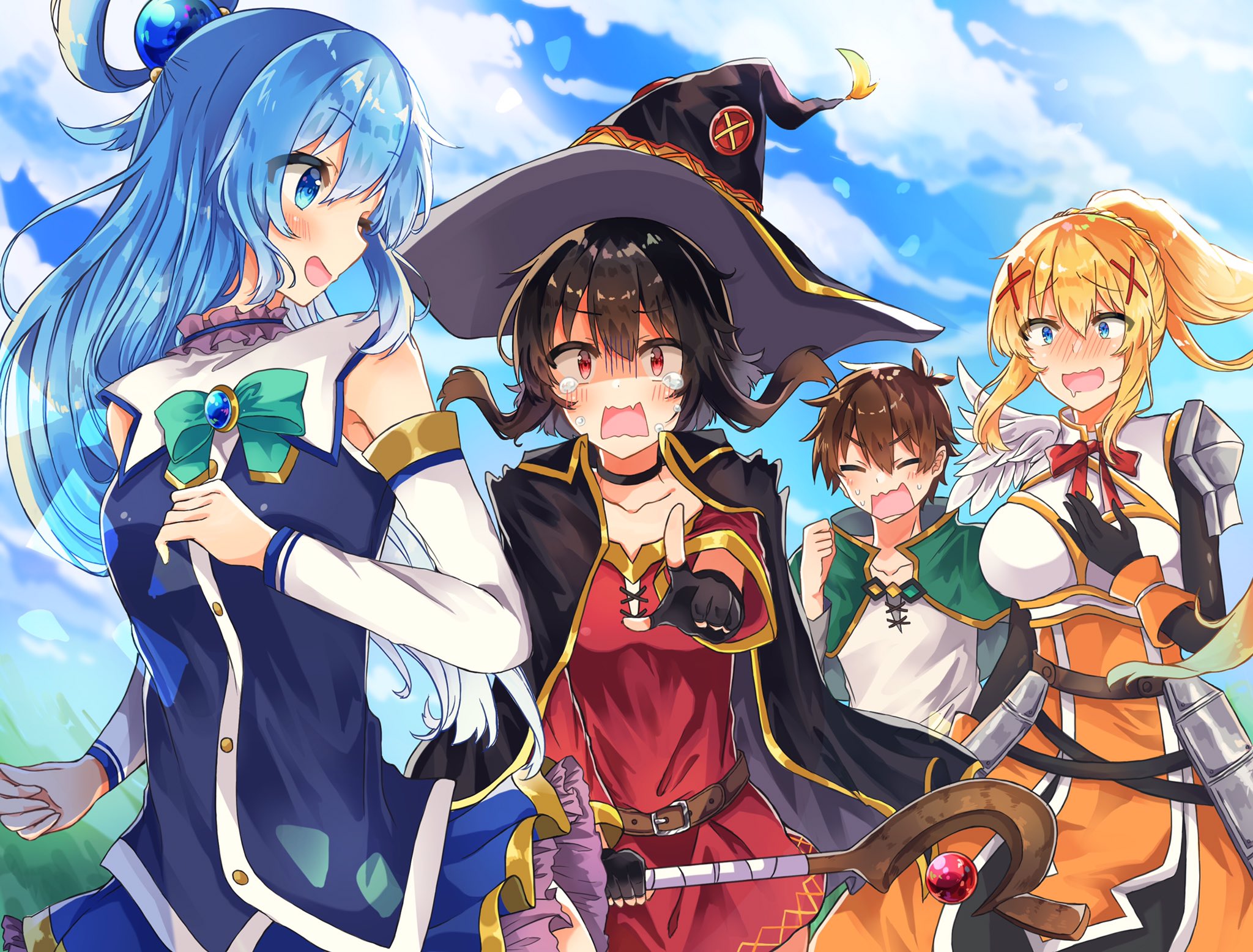