立方体类的设计
- #include <iostream>
- using namespace std;
- class Cub
- {
- private://定义数据
- int m_l;
- int m_w;
- int m_h;
- public:
- //对l w h进行写操作
- void setL(int l)
- {
- m_l = l;
- }
- void setW(int w)
- {
- m_w = w;
- }
- void setH(int h)
- {
- m_h = h;
- }
- //对l w h 进行读操作
- int getL (void)
- {
- return m_l;
- }
- int getW(void)
- {
- return m_w;
- }
- int getH(void)
- {
- return m_h;
- }
- int getS(void)//计算表面积
- {
- return 2*(m_l*m_h+m_l*m_w+m_w*m_h);
- }
- int getV(void)//计算体积
- {
- return m_l*m_w*m_h;
- }
- bool cubCompareInClass(Cub &ob)//成员函数判断
- {
- if(getV() == ob.getV())
- return true;
- else
- return false;
- }
- };
- bool cubCompareInOverall(Cub &c1, Cub &c2)//全局函数
- {
- if(c1.getV() == c2.getV())
- {
- return true;
- }
- return false;
- }
- int main()
- {
- Cub cub1;//实例化对象
- cub1.setH(10);
- cub1.setL(10);
- cub1.setW(10);
- cout << “cub1的面积: ” << cub1.getS() << endl;//计算面积
- cout << “cub1的体积: ” << cub1.getV() << endl;//计算体积
- Cub cub2;//实例化对象
- cub2.setH(10);
- cub2.setL(20);
- cub2.setW(10);
- Cub cub3;//实例化对象
- cub3.setH(10);
- cub3.setL(5);
- cub3.setW(20);
- if(cubCompareInOverall(cub1, cub2) == true)//使用全局函数进行比较
- {
- cout << “体积相等!” << endl;
- }
- else
- {
- cout << “体积不相等!” << endl;
- }
- if(cub3.cubCompareInClass(cub1) == true)//使用成员函数进行比较
- {
- cout << “体积相等!” << endl;
- }
- else
- {
- cout << “体积不相等!” << endl;
- }
- }
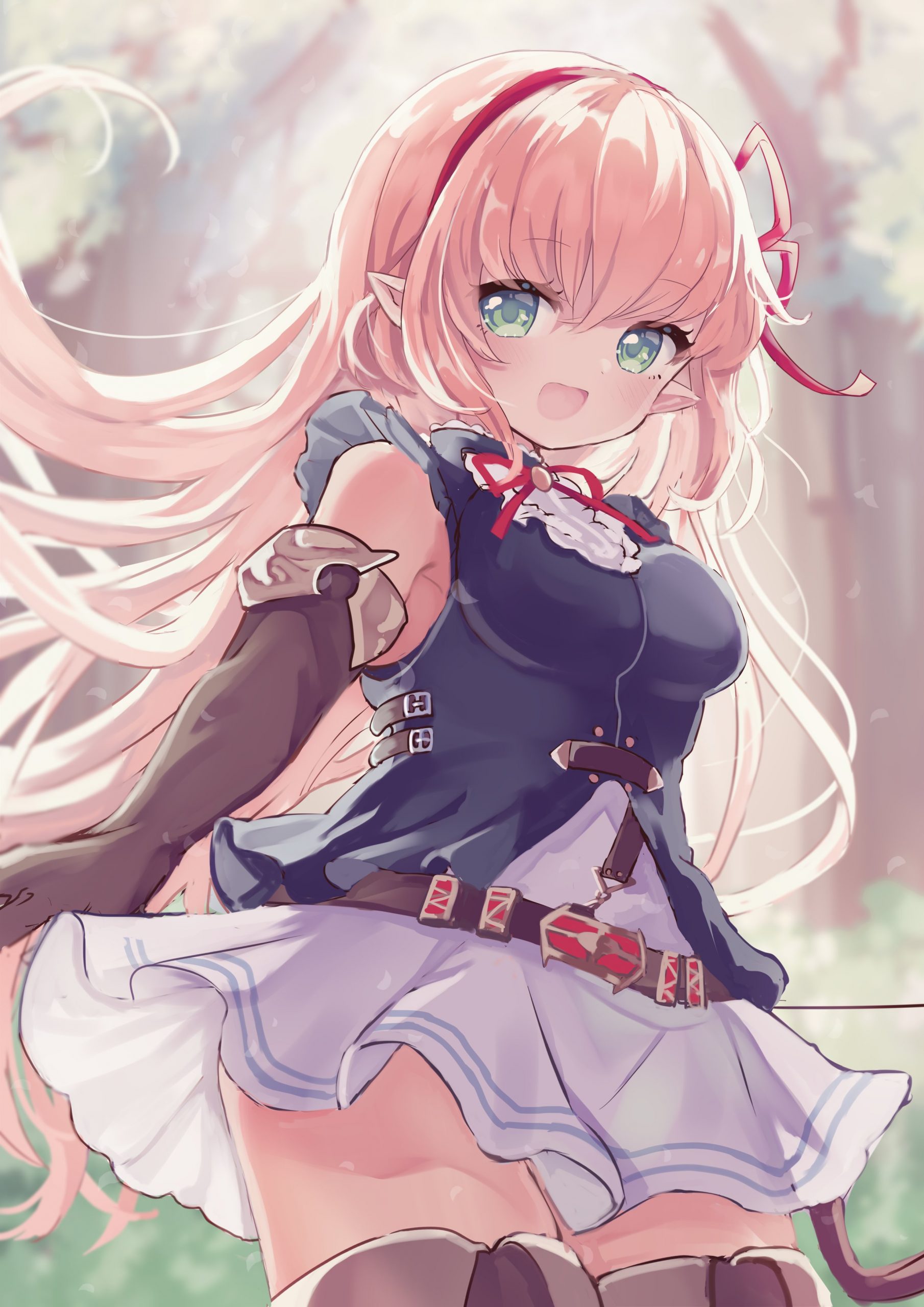